The toolbar script is a plain script file written in
VBScript or JScript (as you specify in the configuration).
It is the place where the main functionality of the IE toolbar
application is implemented.
A separate instance of the toolbar script is loaded
with each visible toolbar. Therefore each Internet Explorer
browser window with your toolbar shown has a separate instance
of this script running. Each instance is initialized when the
toolbar is first shown in that Internet Explorer window and is
unloaded when the window closes. If the user hides/un-hides the
toolbar during the lifecycle of the browser window the toolbar
script is not unloaded, instead it is just advised about the
event (OnShow
event). Thus all the internal variables of the script are
preserved during the lifetime of the window in which the toolbar
is shown.
The toolbar script does its work by sinking events
and doing actions in response to them. The toolbar script has
direct access to the Toolbar user interface area, the
browser work area and to the internal toolbar utilities.
It can create additional objects from the ScriptBar's run-time
library or 3-d party components (using Host.CreateObject)
and thus access a huge set of additional features and ways to
interact with the PC and perform complex tasks.
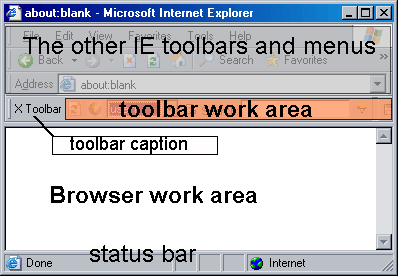
The toolbar script has a set of pre-defined objects
which it can access by using the names listed below at any time.
E.g. the script can call their methods, read/write their
properties whenever needed. These objects are created and
initialized by the newObjects ScriptBar core before the toolbar
script starts and they give it access to the elements (visual
and internal) of the ScriptBar. Through them the toolbar script
is able to: control the visual state of the toolbar's user
interface area, fully control the main work area of the
browser, use the utility routines supplied by the
ScriptBar core, create additional objects for its needs. These
objects are:
Toolbar
A WebBrowser2
object representing the toolbar work area. This object
is implemented by the Microsoft Internet Explorer browser
control which hosts the toolbar's user
interface HTML. This means that the toolbar user interface
and the browser work area are accessed in the same fashion,
only the names of the objects used to reach them are
different.
See WebBrowser2
for details and links to the reference information from
Microsoft.
Example: To access a HTML element in the toolbar work area
with ID "myelement" you use code like this:
Toolbar.document.all("myelement").<element_member>
Browser
A WebBrowser2
object representing the browser work area. his object
is implemented by the Microsoft Internet Explorer browser
control which is hosted in Microsoft Internet Explorer
application and shows pages and other content as the user
browses the WEB. Through this object the toolbar script can
direct the browser to certain URL, determine the current
location, dig into the page/pages currently viewed by the
user, extract content from them or change their
elements.
Example: To access a HTML element in the browser work area
with ID "myelement" you use code like this:
Toolbar.document.all("myelement").<element_member>
Host
Is the object which represents the newObjects ScriptBar
core itself. It provides utility
methods the toolbar script needs in order to perform
vital tasks, create additional objects from the ScriptBar
run-time library, 3-d party objects and thus gain access
to almost all of the features of Microsoft Windows operating
system.
Settings
Is an object that supplies minimal information needed by the
toolbar script to determine its install location and other
parameters that may be needed by the developer in order to
construct an install location independent code.
In the version 1.0 of newObjects ScriptBar there is only one
entry in it which can be accessed this way:
stringvar = Settings("BasePath")
The stringvar receives the full path name of the
install location of the ScriptBar's DLL. This is a directory
path and always ends with "\" character. Thus to
compose the path name in the same directory it is enough to
concatenate the file name to it:
filepath = Settings("BasePath") & "myfile.txt"
This allows the toolbar script find and use any additional
resource files needed for its work, for example database
files, custom configurations, log files and so on.
To access various other features supplied by the run-time
libraries and 3-d party components the toolbar script uses Host.CreateObject
method to create certain object and saves it to a script
variable. Then the created object is used to call methods,
read/write properties on it. The newObjects ScriptBar's run-time
library contains a rich set of objects. They all ship
with the toolbar and thus they are always available on the
system on which it is installed. It is strongly recommended to
use the objects from the ScriptBar's run time library instead of
any other 3-d party object whenever the required feature is
supplied by them. This will keep the final product easy for
re-distribution and will guarantee its compatibility with all
the supported operating system versions. If a 3-d party
component is a must the developer must take care to
re-distribute its DLL(s) with the final product or/and implement
system check for their availability prior or during the
installation process.
A simple example code which illustrates some of the
information on this page is shown below. More sample code
snippets can be found on the pages describing the different
objects and fully working examples can be downloaded from our
site. A few words about the sample's functionality can be read
below it on this page.
The toolbar script file (in VBScript)
The names of the pre-defined objects used in this sample are
bolded and the event handler routine is bold-itallic.
' This code is in VBScript, but it can be written in JScript as well
' This snippet looks for certain words in the
Const cSFShareDenyNone = &H00000040
Const cReadLineAny = -3
Dim sf, words, file
' Read the words/expressions from the file
Set sf = Host.CreateObject("newObjects.utilctls.SFMain")
Set words = Host.CreateObject("newObjects.utilctls.VarDictionary")
words.allowUnnamedValues = True
Set file = sf.OpenFile(Settings("BasePath") & "words.txt",cSFShareDenyNone)
' The file contans one word/expression per line.
' We load them into a dictionary object and we make them upper case
' to make the search a bit more easier below
Dim line
While Not file.EOS
line = Trim(file.ReadText(cReadLineAny))
If line <> "" Then
words.Add "", UCase(line)
End If
Wend
' We handle this event which is fired whenever a new page opens in the
' browser work area. And we search that page for the words loaded during
' the toolbar initialization
Sub OnDocumentComplete(frame,url)
Dim I
Dim docContent
Dim str
str = ""
docContent = frame.document.body.innerText
For I = 1 To words.Count
If InStr(UCase(docContent),words(I)) > 0 Then
If str <> "" Then str = str & ", "
str = str & words(I)
End If
Next
Toolbar.document.all("Found").innerText = str
Toolbar.document.all("Found").title = url
End Sub
The toolbar user interface HTML.
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=windows-1251">
<title>Toolbar</title>
<BASE HREF="%BASE%">
<SCRIPT>
</SCRIPT>
<STYLE>
body { font-family: Arial; font-size: 11px }
td { font-family: Arial; font-size: 11px }
</STYLE>
</head>
<body bgcolor="buttonface" text="buttontext" topmargin="0" leftmargin="0" SCROLL="NO">
<TABLE WIDTH="100%" HEIGHT="100%">
<TR>
<TD VALIGN="Middle" nowrap WIDTH="100%">
<B><SPAN ID="Found"> </SPAN></B>
</TD>
</TR>
</TABLE>
</body>
</html>
The contents of the words.txt file used to try the sample
(see the snapshot below):
Windows
system
drivers
software
components
DLL
developer
computer
And a snapshot of the sample while the browser shows a
page from our site.
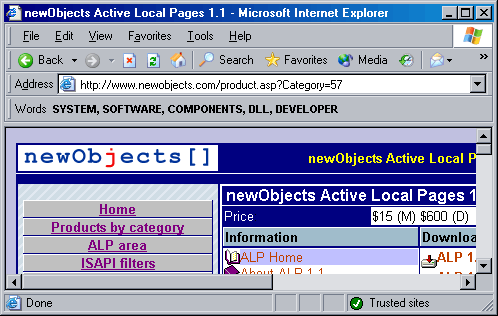
Comments
This basic sample uses the toolbar as a simple display area.
It loads a text file with a word/expression on each line and
searches those words/expressions in each page viewed by the
user. The words found are listed in the toolbar's work area.
Assume that it is a kind of reminder for the user - informing
him/her that the page may contain something of interest.
Of course, this is just a sample. A real-world application
should be more precise and more flexible. For example SQLite
COM database can be used for the words, some buttons/images
can be on the toolbar to allow user enable/disable it, a precise
logic can be used to identify the frames (if the page is a
frameset) and may be display the results grouped by frame and so
on.
|